You've probably experienced it before: you want to return multiple elements in a render()
method so you're forced to wrap them in a <span>
or <div>
element which will go unused. In comes the React.Fragment
component to solve this issue – let's take a quick look at some examples!
Before
Using this syntax...
render() {
return (
<div>
<h1>Todo Title</h1>
<p>Feed the baby</p>
</div>
)
}
Will result in the div
being added to our dom...
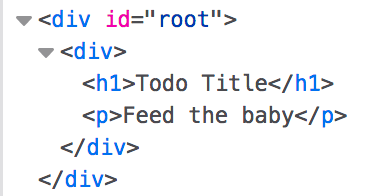
After
However, using the React Fragment syntax...
render() {
return (
<React.Fragment>
<h1>Todo Title</h1>
<p>Feed the baby</p>
</React.Fragment>
)
}
Means we have a valid component but without the unused element in the dom...
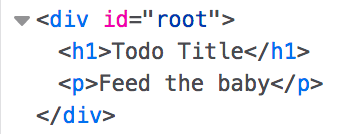
You can see how this would come in handy as your components grow with your application. And starting in v16.2.0
, React took Fragments a step further with shorthand syntax:
render() {
return (
<> {/* So short, you might even miss it's there! */}
<h1>Todo Title</h1>
<p>Feed the baby</p>
</>
)
}
Cheers to less typing and more succinct code!